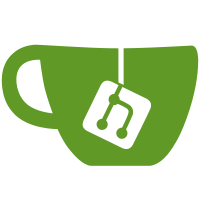
[5] **WORKING** add custom item and item group to game. primarily metaljacket items not added yet - but example item works. [5] initial add of custom blocks [5] Made adding blocks more dynamic - tested to work [5] Fixed metal jacket armor & created correct texture files to not break game. TEXTURES ARE RANDOM ON WEARING AS I DONT HAVE AN ACTUAL TEXTURE YET [5] Started attribute tree stuff
244 lines
9.1 KiB
Java
244 lines
9.1 KiB
Java
/*
|
|
*
|
|
* ConfigManager
|
|
*
|
|
* This class is the central configuration file manager for all other classes and acts as a utility class for other classes to use.
|
|
* It is typical to define this class as a general object (not static instance) for each class in single-threaded actions. If you need
|
|
* a special function or action from this class; it is recommended to add it to this class and not make your own.
|
|
*
|
|
*
|
|
*/
|
|
|
|
package jesse.keeblarcraft.ConfigMgr;
|
|
|
|
import java.io.FileWriter;
|
|
import java.io.File;
|
|
import java.io.FileNotFoundException;
|
|
import java.io.IOException;
|
|
|
|
import com.google.common.base.Charsets;
|
|
import com.google.common.io.Files;
|
|
import com.google.gson.Gson;
|
|
import com.google.gson.GsonBuilder;
|
|
import com.google.gson.JsonIOException;
|
|
import com.google.gson.JsonSyntaxException;
|
|
|
|
import java.util.List;
|
|
|
|
import org.apache.commons.io.FileUtils;
|
|
|
|
import java.util.ArrayList;
|
|
|
|
import jesse.keeblarcraft.Utils.ChatUtil;
|
|
import jesse.keeblarcraft.Utils.ChatUtil.CONSOLE_COLOR;
|
|
import jesse.keeblarcraft.Utils.CustomExceptions.*;
|
|
|
|
public class ConfigManager {
|
|
|
|
// Pedantic empty constructor
|
|
public ConfigManager() {}
|
|
|
|
// CreateFile
|
|
//
|
|
// Returns true if file is created, will return false if file cannot be created (returns true if already exists)
|
|
public Boolean CreateFile(String fileName) throws FILE_CREATE_EXCEPTION {
|
|
Boolean ret = false;
|
|
File file = new File(fileName);
|
|
|
|
// Check 1: Does the file already exist?
|
|
ret = file.exists();
|
|
System.out.println(ChatUtil.ColoredString("Does file exist? ", CONSOLE_COLOR.BLUE) + (ret ? ChatUtil.ColoredString("YES", CONSOLE_COLOR.YELLOW) : ChatUtil.ColoredString("NO", CONSOLE_COLOR.YELLOW)));
|
|
|
|
// Check 2: If the file does not exist, attempt to create it
|
|
if (ret == false) {
|
|
try {
|
|
ret = file.createNewFile();
|
|
} catch (IOException e) {
|
|
// The file could not be created
|
|
throw new FILE_CREATE_EXCEPTION();
|
|
}
|
|
} else {
|
|
ret = true; // This might be a hot fix, but technically the file already exists so would this be true or false? --?this statement is wild?
|
|
System.out.println(ChatUtil.ColoredString("File (name: ", CONSOLE_COLOR.BLUE) + ChatUtil.ColoredString(fileName, CONSOLE_COLOR.YELLOW) + ChatUtil.ColoredString(" was determined to already exist. Exiting out", CONSOLE_COLOR.BLUE));
|
|
}
|
|
return ret;
|
|
}
|
|
|
|
// DeleteFile
|
|
//
|
|
// Returns true if file is deleted, false if could not be deleted or does not exist
|
|
public Boolean DeleteFile(String fileName) throws FILE_DELETE_EXCEPTION {
|
|
Boolean ret = false;
|
|
File file = new File(fileName);
|
|
|
|
// Step 1: Does file exist?
|
|
ret = file.exists();
|
|
|
|
// Step 2: If file exists, attempt to delete
|
|
if (ret == true) {
|
|
try {
|
|
ret = file.delete();
|
|
} catch (SecurityException e) {
|
|
throw new FILE_DELETE_EXCEPTION();
|
|
}
|
|
} else {
|
|
System.out.println(ChatUtil.ColoredString("cannot delete file ", CONSOLE_COLOR.RED) + ChatUtil.ColoredString(fileName, CONSOLE_COLOR.YELLOW) + ChatUtil.ColoredString(" because file does not exist", CONSOLE_COLOR.BLUE));
|
|
}
|
|
return ret;
|
|
}
|
|
|
|
// WriteToFile
|
|
//
|
|
// Will write or append to file (valid modes: "w" or "a") if file is available. Returns false if not
|
|
public Boolean WriteToFile(String fileName, String data, String mode) {
|
|
Boolean ret = false;
|
|
|
|
FileWriter file;
|
|
try {
|
|
file = new FileWriter(fileName);
|
|
switch(mode) {
|
|
case "w":
|
|
file.write(data);
|
|
ret = true;
|
|
break;
|
|
case "a":
|
|
file.append(data);
|
|
ret = true;
|
|
break;
|
|
default:
|
|
System.out.println(ChatUtil.ColoredString("Invalid mode to WriteToFile!", CONSOLE_COLOR.RED));
|
|
break;
|
|
}
|
|
|
|
file.close();
|
|
} catch (IOException e) {
|
|
System.out.println(ChatUtil.ColoredString("Could not open file ", CONSOLE_COLOR.RED) + ChatUtil.ColoredString(fileName, CONSOLE_COLOR.YELLOW) + ChatUtil.ColoredString(" to write to it! Possibly permission issue?", CONSOLE_COLOR.RED));
|
|
}
|
|
|
|
return ret;
|
|
}
|
|
|
|
|
|
// WriteToJsonFile
|
|
//
|
|
// Will write to or append to a json file. It will search if the key exists first & update the field;
|
|
// or add a new entry. It should be noted that json objects *can* be buried inside each other. It is
|
|
// considered best (and only) practice to call the "GetJsonStringFromFile" function first from this
|
|
// class and simply iterate to what you would need and then update the entire entry alongside the
|
|
// top-level key.
|
|
//
|
|
// NOTE: THIS DOES NOT SAFE UPDATE THE KEY OBJECT. PRE-EXISTING DATA WILL BE DELETED FOREVER
|
|
public void WriteToJsonFile(String fileName, Object data) throws FILE_WRITE_EXCEPTION {
|
|
Gson gson = new GsonBuilder().setPrettyPrinting().create();
|
|
try {
|
|
FileWriter writer = new FileWriter(fileName);
|
|
gson.toJson(data, writer);
|
|
writer.flush();
|
|
writer.close();
|
|
} catch (JsonIOException | IOException e) {
|
|
System.out.println(ChatUtil.ColoredString("Could not successfully write to json file", CONSOLE_COLOR.RED));
|
|
throw new FILE_WRITE_EXCEPTION();
|
|
}
|
|
}
|
|
|
|
// GetJsonStringFromFile
|
|
//
|
|
// Retrieves json file and converts to desired object. Returns JsonSyntaxException if file could not be fitted to class input
|
|
public <T> T GetJsonObjectFromFile(String fileName, Class<T> classToConvertTo) throws JsonSyntaxException {
|
|
Gson gson = new Gson();
|
|
String ret = "";
|
|
|
|
// hot fix: Not sure how to return "false" for invalid conversion when I'm forced to convert or just catch... Look into a better
|
|
// return value in the future - but for now throw JsonSyntaxException no matter what exception is caught
|
|
try {
|
|
File file = new File(fileName);
|
|
ret = FileUtils.readFileToString(file, "UTF-8");
|
|
} catch (NullPointerException e) {
|
|
System.out.println(ChatUtil.ColoredString("nullptr exception", CONSOLE_COLOR.RED));
|
|
throw new JsonSyntaxException("");
|
|
} catch (FileNotFoundException e) {
|
|
System.out.println(ChatUtil.ColoredString("file not found", CONSOLE_COLOR.RED));
|
|
throw new JsonSyntaxException("");
|
|
} catch (java.nio.charset.UnsupportedCharsetException e) {
|
|
System.out.println(ChatUtil.ColoredString("charset issue", CONSOLE_COLOR.RED));
|
|
throw new JsonSyntaxException("");
|
|
} catch (IOException e) {
|
|
System.out.println(ChatUtil.ColoredString("io exception", CONSOLE_COLOR.RED));
|
|
throw new JsonSyntaxException("");
|
|
}
|
|
|
|
return gson.fromJson(ret, classToConvertTo);
|
|
}
|
|
|
|
public Boolean DoesFileExist(String fileName) {
|
|
Boolean ret = false;
|
|
|
|
return ret;
|
|
}
|
|
|
|
public Boolean DoesDirectoryExist(String dirName) {
|
|
Boolean ret = false;
|
|
|
|
return ret;
|
|
}
|
|
|
|
public Boolean CreateDirectory(String dirName) throws DIRECTORY_CREATE_EXCEPTION {
|
|
Boolean ret = false;
|
|
|
|
File dir = new File(dirName);
|
|
|
|
try {
|
|
if ( ! dir.exists()) {
|
|
ret = dir.mkdirs();
|
|
}
|
|
} catch (Exception e) {
|
|
System.out.println(ChatUtil.ColoredString("Failed to make directory with name: ", CONSOLE_COLOR.RED) + ChatUtil.ColoredString(dirName, CONSOLE_COLOR.YELLOW));
|
|
ret = true; /// TODO: Hack to make Setup fn be fine with prev-created files! Make Setup more robust!
|
|
throw new DIRECTORY_CREATE_EXCEPTION();
|
|
}
|
|
return ret;
|
|
}
|
|
|
|
public Boolean DeleteDirectory(String dirName) throws DIRECTORY_DELETE_EXCEPTION {
|
|
Boolean ret = false;
|
|
|
|
File dir = new File(dirName);
|
|
|
|
try {
|
|
ret = dir.delete();
|
|
System.out.println(ChatUtil.ColoredString("Deleted directory ", CONSOLE_COLOR.GREEN) + ChatUtil.ColoredString(dirName, CONSOLE_COLOR.YELLOW));
|
|
} catch (Exception e) {
|
|
System.out.println(ChatUtil.ColoredString("Failed to delete directory: ", CONSOLE_COLOR.RED) + ChatUtil.ColoredString(dirName, CONSOLE_COLOR.YELLOW));
|
|
throw new DIRECTORY_DELETE_EXCEPTION();
|
|
}
|
|
|
|
return ret;
|
|
}
|
|
|
|
// AddToKey
|
|
//
|
|
// Adds new text to a key if found inside the config
|
|
public String AddToKey(String key, String newInfo) {
|
|
String ret = "";
|
|
|
|
return ret;
|
|
}
|
|
|
|
// GetFile
|
|
//
|
|
// Returns a file as an arraylist of all the lines in the file. Generally only used for testing
|
|
//
|
|
// NOTE: Returns UTF-8 Encoding of file
|
|
public List<String> GetFile(String fileName) {
|
|
List<String> ret = new ArrayList<String>();
|
|
|
|
try {
|
|
return Files.readLines(new File(fileName), Charsets.UTF_8);
|
|
} catch (IOException e) {
|
|
ret.clear();
|
|
}
|
|
|
|
return ret;
|
|
}
|
|
}
|